Test Automation
Selenium WebDriver
TestNG
POM
(See test case examples below)
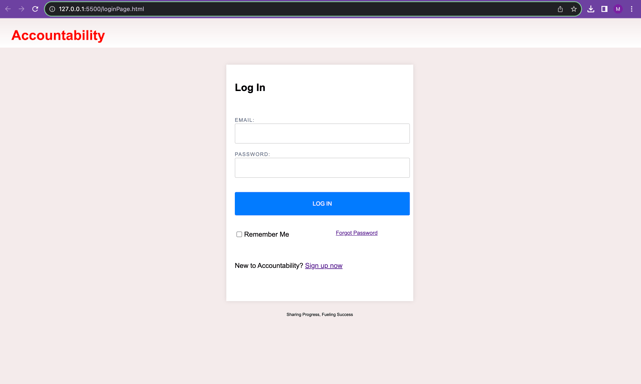
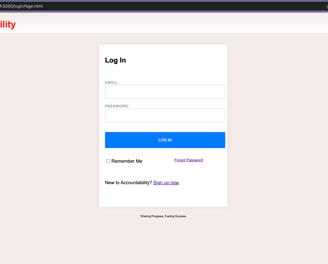
Test Cases for Login Page:
Valid User Login:
Verify that a user can successfully log in with a valid username and password.
Ensure the user is redirected to the appropriate landing page after a successful login.
Invalid User Login:
Test login with an invalid username and a valid password.
Test login with a valid username and an invalid password.
Verify that appropriate error messages are displayed for invalid login attempts.
Empty Fields:
Test login without entering any username or password and ensure it's not allowed.
Verify that error messages are displayed for both empty fields.
Password Masking:
Ensure that the password field masks characters (e.g., asterisks or dots) for security purposes.
Case Sensitivity:
Test the case sensitivity of the username and password fields (e.g., ensure that "User" and "user" are treated as different inputs).
Remember Me:
Test the "Remember Me" checkbox to ensure it remembers the user's login across sessions.
Session Timeout:
Test the session timeout functionality by leaving the page idle for an extended period and verifying that the user is automatically logged out.
Forgot Password:
Test the "Forgot Password" link and ensure that users can reset their passwords via email.
Account Lockout:
Test account lockout functionality after a specified number of failed login attempts.
Verify that locked-out users receive an email notification and can unlock their accounts.
Security and Validation:
Test for security vulnerabilities, such as SQL injection and cross-site scripting (XSS) attacks.
Ensure that input fields are properly validated to prevent malicious input.
Password Strength:
Verify that the system enforces password strength rules (e.g., minimum length, special characters, numbers, etc.).
Browser Compatibility:
Test the login page on different web browsers (e.g., Chrome, Firefox, Safari, Edge) to ensure compatibility.
Mobile Responsiveness:
Test the login page on various mobile devices (e.g., smartphones, tablets) to ensure responsive design.
Localization:
Test the login page with different languages and character sets to ensure text and error messages are displayed correctly.
Performance:
Test the login page's performance under load to ensure it can handle concurrent login attempts without issues.
Accessibility:
Verify that the login page is accessible to users with disabilities by testing it with screen readers and keyboard navigation.
Cross-browser and Cross-platform Testing:
Test the login page on different operating systems (e.g., Windows, macOS, Linux) to ensure compatibility.
Browser Developer Tools Testing:
Test the login page using browser developer tools to inspect network requests, check for console errors, and debug issues.
User Experience (UX) Testing:
Evaluate the overall user experience of the login page, including user-friendly error messages and clear instructions.
Edge Cases:
Test edge cases such as very long usernames or passwords to ensure the system handles them gracefully.